Closing the Gaps in Dev Workflows: RPAs as the Missing Automation Layer
Developers waste precious time switching between tools, creating death-by-micro-interruptions. To solve this, we built a browser-based RPA framework to automate our daily workflows. Here’s how.

Any software engineer can tell you: writing code is only half the job. And the other half? A relentless shuffle of tools, hotkeys, dashboards, and digital duct tape holding the day together.
One moment you’re deep in logic, and the next you’re hunting for a build log, checking Slack threads, copy-pasting ticket IDs, or untangling a broken integration. We’re not just software engineers; we’re part-time database admins, CI detectives, dashboard archaeologists, and accidental project managers.
While each of these tasks might feel insignificant, the constant switching chips away at our momentum. We lose the thread. We lose time. We lose patience. It’s death by a thousand micro-interruptions.
What’s worse - it isn’t just a personal productivity issue. It’s a team-wide slowdown. Every repeated manual step, every brittle process, every confusing tool adds friction. And when that friction compounds across an entire engineering organization, it’s not just minutes lost, it’s entire sprints dragged down.
But what if we could spot where time was leaking out? Maybe we could build something to fix it. Spoiler alert: that’s exactly what we did. And the best part is we didn’t need a fancy audit to help us spot the inefficiencies. All we needed was to pay closer attention to our own workflows.
Introducing Automation into Developer Workflow
New engineers were the first clue. Every time they paused, asked a question, or backtracked during onboarding, we saw the system through fresh eyes. We treated those pauses as signals that something was off.
Then we tracked every time we switched tools, hit a speed bump, or retyped the same thing twice, and we asked ourselves: Do we actually need this step? Could this be automated? And why isn’t the relevant data already in the UI?
That’s when it became clear we’d been sitting on a hidden advantage.
Once we realized that we were jumping between the same few tools – CI dashboards, GitHub, Slack, ticketing systems – and manually stitching them together over and over again, something clicked.
It was begging to be automated.
And we knew right away we didn’t want to simply write dozens of patchwork scripts. Instead, we stepped back and designed something way more robust.
Island makes it easy to update and deliver robotic process automations (RPAs) to whole teams. RPAs live in a central management console, and upgrade with a single click. Admins enable an RPA on a policy rule, and the change rolls out instantly.
We built a single RPA tailored to an entire website that watches the browser’s URL and runs the right automation based on pattern matching—kind of like how a front-end app shows different views without reloading the page. A URL route can be registered with its own set of small, focused features. Every feature is a self-contained function – isolated, safe from double-injection, and designed to run independently.
// router.ts
export class Router {
routes: { pattern: RegExp; callback: () => Promise<void> }[] = [];
add(pattern: RegExp, callback: () => Promise<void>) {
this.routes.push({ pattern, callback });
}
async run() {
const url = window.location.href;
for (const { pattern, callback } of this.routes) {
if (pattern.test(url)) {
console.log(`Matched: ${pattern}`);
await callback();
return;
}
}
console.log("No route matched.");
}
}
// main.ts
import { Router } from "./router";
import { createSidebar } from "./createSidebar";
const router = new Router();
router.add(/^https:\/\/github\.com\/.*\/pull\/\d+$/, async () => {
const config = [
{
title: "CI",
settings: [
{ name: "Hide Passing CI", fn: () => {/* hide passing ci logic */} },
{ name: "Hide Skipped CI", fn: () => {/* hide skipped ci logic */} },
],
},
// more groups...
];
createSidebar(config);
});
window.addEventListener("load", () => {
router.run();
});
// createSidebar.ts
export function createSidebar(config) {
for (const group of config) {
group.settings.forEach((setting) => {
const enabled = localStorage.getItem(setting.name) === "true";
if (enabled) setting.fn();
const checkbox = document.createElement("input");
checkbox.type = "checkbox";
checkbox.checked = enabled;
checkbox.onchange = () => {
localStorage.setItem(setting.name, checkbox.checked.toString());
if (checkbox.checked) setting.fn();
};
document.body.appendChild(checkbox);
document.body.appendChild(document.createTextNode(setting.name));
});
}
}
Developers can toggle features on or off via local storage, giving each person the ability to customize their own workflow, enabling just the enhancements they want – without changing anything for others.
This structure isn’t just flexible, it’s collaborative. RPA doesn’t live with a single expert or gatekeeper. Instead, it grows through contributions from the whole team, with everyone free to build, refine, and share new features.
Now instead of automation being a one-off task, it’s a platform. One that sits invisibly in the browser, detects where you are, and quietly brings the right functionality with it – whether that’s restarting a build, preloading a Slack message, or adding real-time timestamps to a noisy dashboard.
It feels less like automation, and more like building our own personal dev tools right into the browser.
A Look at Island’s RPA Platform in Action
Below are a few ways our RPA framework has started streamlining day-to-day tasks:
1. One-Click CI Restart
The quintessential feature. No more digging through Jenkins or GitHub Actions. Instead, trigger an RPA shortcut to restart your build. This alone can save the frustration of multiple clicks and screen loads in a typical CI/CD interface.

2. Slack Links for Code Reviews
Automatically identify and list the relevant Slack channels for a particular project or repository when the code reviewers list changes. It also adds a link to the PR in your clipboard. Click the channel name to open it in Slack with the PR link ready to paste.
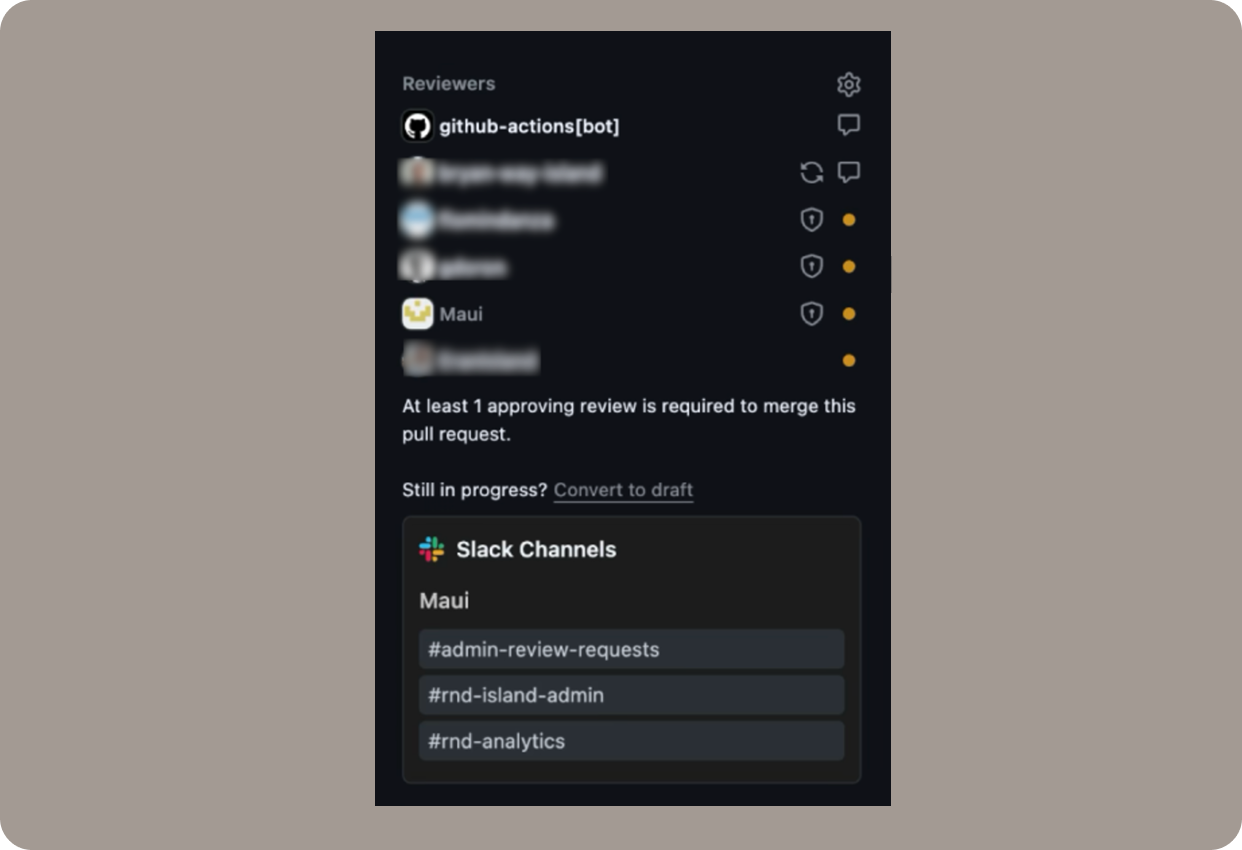
3. Decluttering the Timeline
Filter out bot messages, old threads, and random noise from your timeline. By auto-hiding irrelevant messages or grouping them into an “FYI only” category, it’s easier to focus on what truly matters.
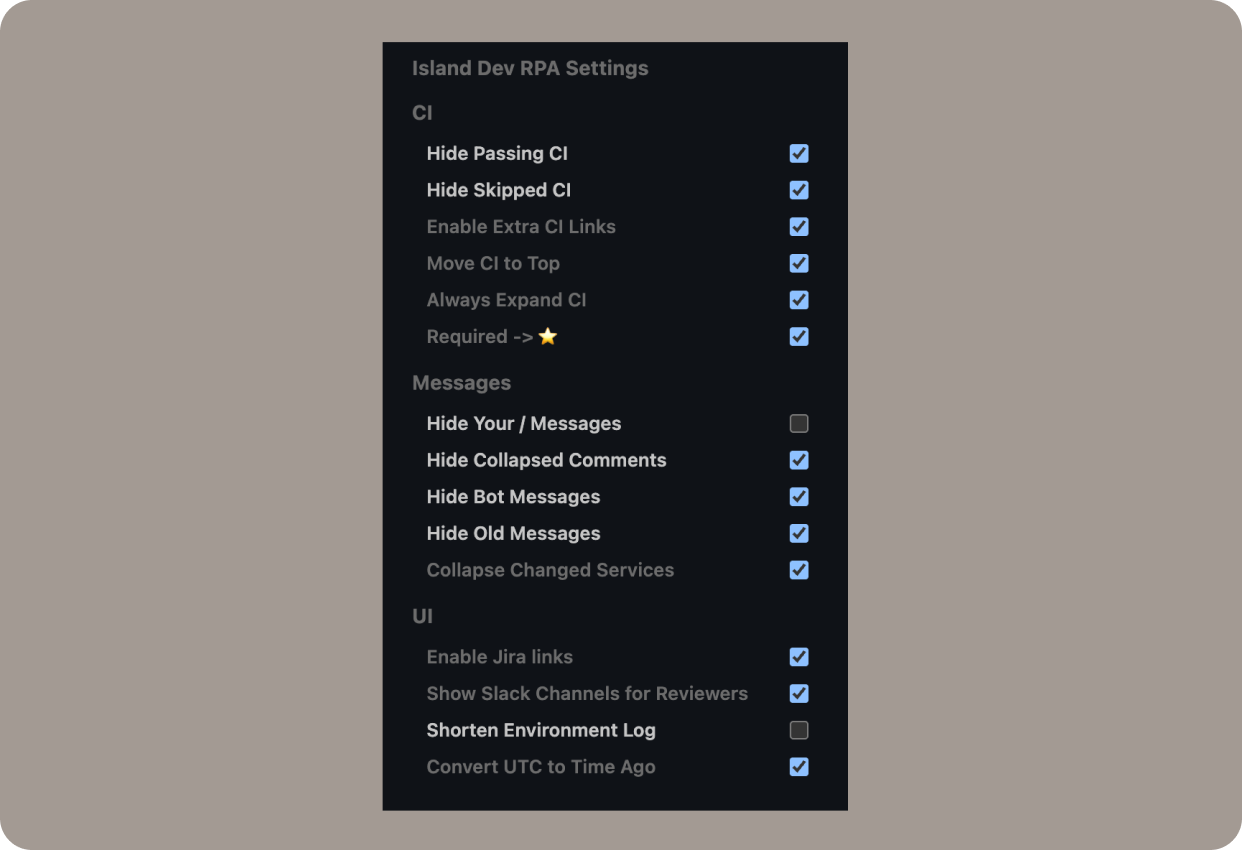
4. Human-Readable Times
Convert UTC timestamps into “X days ago” or “X hours ago.” This minor tweak can have a big impact when sifting through logs, build histories, or messages, making timelines more intuitive. For example, a lot of automatic output from Jenkins to GitHub might post things in UTC format. It's not dynamic text and there's not really a better way to change this. But with an RPA, we can identify those pieces of text and convert them to local time and even better, convert them to "time ago" format. Now all these hard to read times even update in real time.

5. Jenkins Console Navigation Map
Large, nested build outputs can be a maze. Our automated “navigation map,” inspired by VSCode, helps you jump directly to failing steps or relevant lines. We highlight errors and important links, and skip known phrases or keywords that you want to ignore, trimming valuable minutes off each debug session.
Taken together, these small improvements add up, chipping away at the daily friction developers face. They also encourage more consistent best practices – because when the easiest way to do something is also the right way, everyone wins.

The Future of RPA in Engineering
When we first started building these automations, we weren’t thinking about the future. We were thinking about surviving the week by shaving five minutes off a task we did ten times a day. But as our scripts evolved, so have our perspectives.
Each time we automated a frustrating step – copying links, digging through logs, navigating brittle dashboards – it brought us back a little more focus. And now that we’ve seen what that focus is worth, we want more of it.
So as we begin to imagine: what if the browser could do more than just assist? What if it could anticipate? This is where things really start to get interesting.
By combining RPA with AI, we see a future where the browser doesn’t just carry out tasks; it helps shape them. It can spot patterns in failing CI builds, suggest what to automate next, and maybe even write the first draft of the script for you.
This is not science fiction. It’s a natural extension of the tools we’re already building. The more we offload mechanical, repetitive work, the more we get to focus on the parts of software engineering that actually feel like engineering – creative problem-solving, high-level design, and building things that matter.
How Does the Framework Scale?
All of these possibilities came through trial and error, and over time we found a rhythm – a set of principles that help us scale without losing our way.
Keep it lightweight
The moment a script becomes annoying – too noisy, too pushy – it stops being helpful. By keeping features modular and narrowly scoped, we avoid the bloat that drags systems down.
Let engineers customize
No two workflows are the same. That’s why every script comes with sensible defaults, but is also easy to tweak. When developers can tune things to their needs, adoption happens naturally.
Spread ownership
RPA isn’t owned by one person or one team. Anyone can build, improve, or refine a feature. That kind of distributed model means faster iteration and deeper buy-in.
Measure what matters
We track which scripts are actually being used, and which ones are just taking up space. That feedback loop helps us trim what doesn’t work and double down on what does.
Not only have these principles helped us scale internally, but they’ve also opened doors to collaboration with QA, DevOps, and support – with each team bringing their own ideas, friction points, and fixes.
We’ve seen first-hand how small, targeted automation can scale across teams. We’ve learned how to keep it flexible, how to make it sustainable, and how to build momentum without creating overhead.
But at the core of it all, this isn’t just about scripts or workflows.
It’s about focus.
In Conclusion
What began as a quiet, underused feature in our enterprise browser became a flexible framework that empowers every developer on the team. And as it spreads across teams and tools, it keeps showing us just how much friction we’ve been tolerating without even realizing it.
Clearing away the daily drag helps us stay focused on the work that truly matters. Small pain points solved early opens the door to bigger wins down the road. And with RPA as a shared capability – not a burden – we’re building workflows that scale with our ambition.
The Island Enterprise Browser fundamentally transforms how the world’s leading organizations work by embedding enterprise-grade IT, security, network controls, data protections, app access, and productivity enhancements directly into the browser itself, enabling secure access to any application, protecting sensitive data, streamlining IT operations, and delivering a superior end-user experience while actually boosting productivity.
To learn more about how we're reimagining the enterprise workspace from the browser up, start here.
If you’re interested in building something that’s changing everything, check out our open positions here.